How To Add List To A Set In Python
A set is an unordered collection of items. Every set chemical element is unique (no duplicates) and must exist immutable (cannot exist changed).
All the same, a ready itself is mutable. We can add or remove items from it.
Sets can also exist used to perform mathematical set operations like matrimony, intersection, symmetric divergence, etc.
Creating Python Sets
A set is created by placing all the items (elements) inside curly braces {}
, separated by comma, or past using the built-in prepare()
function.
Information technology can have whatsoever number of items and they may exist of different types (integer, bladder, tuple, string etc.). Only a set cannot have mutable elements like lists, sets or dictionaries every bit its elements.
# Dissimilar types of sets in Python # ready of integers my_set = {1, 2, 3} print(my_set) # set of mixed datatypes my_set = {i.0, "Hi", (one, 2, three)} impress(my_set)
Output
{one, 2, 3} {1.0, (1, 2, 3), 'Hello'}
Try the following examples as well.
# set cannot have duplicates # Output: {1, two, 3, four} my_set = {one, two, three, four, 3, 2} impress(my_set) # we can brand gear up from a list # Output: {1, ii, 3} my_set = set([1, 2, 3, ii]) impress(my_set) # fix cannot have mutable items # here [3, 4] is a mutable list # this will cause an error. my_set = {ane, 2, [iii, 4]}
Output
{ane, 2, 3, 4} {1, 2, iii} Traceback (most recent telephone call last): File "<cord>", line fifteen, in <module> my_set = {1, two, [3, 4]} TypeError: unhashable blazon: 'list'
Creating an empty fix is a bit catchy.
Empty curly braces {}
volition make an empty dictionary in Python. To make a set without any elements, we use the set()
function without any argument.
# Distinguish gear up and dictionary while creating empty set # initialize a with {} a = {} # check data blazon of a print(blazon(a)) # initialize a with gear up() a = set() # bank check data blazon of a print(blazon(a))
Output
<class 'dict'> <class 'set'>
Modifying a gear up in Python
Sets are mutable. Nevertheless, since they are unordered, indexing has no meaning.
We cannot access or modify an chemical element of a set up using indexing or slicing. Gear up data type does non back up information technology.
We can add a single chemical element using the add together()
method, and multiple elements using the update()
method. The update()
method tin can have tuples, lists, strings or other sets as its argument. In all cases, duplicates are avoided.
# initialize my_set my_set = {1, 3} print(my_set) # my_set[0] # if y'all uncomment the above line # you lot will get an error # TypeError: 'set' object does non support indexing # add together an element # Output: {1, 2, 3} my_set.add(two) print(my_set) # add multiple elements # Output: {1, 2, iii, four} my_set.update([2, 3, four]) print(my_set) # add together list and set # Output: {one, 2, 3, 4, 5, 6, eight} my_set.update([4, v], {1, half-dozen, 8}) print(my_set)
Output
{1, 3} {1, 2, 3} {1, 2, 3, 4} {1, 2, 3, four, 5, 6, eight}
Removing elements from a set
A detail item can be removed from a set using the methods discard()
and remove()
.
The just difference between the two is that the discard()
function leaves a set unchanged if the element is not nowadays in the set. On the other hand, the remove()
function will enhance an error in such a condition (if chemical element is non present in the set).
The post-obit example will illustrate this.
# Difference between discard() and remove() # initialize my_set my_set = {1, iii, 4, 5, 6} print(my_set) # discard an chemical element # Output: {1, iii, 5, 6} my_set.discard(iv) print(my_set) # remove an element # Output: {1, 3, 5} my_set.remove(vi) impress(my_set) # discard an element # non present in my_set # Output: {ane, iii, 5} my_set.discard(2) print(my_set) # remove an element # non nowadays in my_set # you will become an error. # Output: KeyError my_set.remove(ii)
Output
{1, 3, 4, 5, 6} {i, 3, 5, vi} {one, three, v} {1, three, 5} Traceback (most contempo call last): File "<string>", line 28, in <module> KeyError: 2
Similarly, we tin can remove and return an particular using the pop()
method.
Since set is an unordered information type, there is no way of determining which item will be popped. Information technology is completely arbitrary.
We can also remove all the items from a prepare using the clear()
method.
# initialize my_set # Output: ready of unique elements my_set = set("HelloWorld") print(my_set) # pop an element # Output: random element impress(my_set.pop()) # pop some other element my_set.pop() print(my_set) # clear my_set # Output: set() my_set.clear() print(my_set)
Output
{'H', '50', 'r', 'W', 'o', 'd', 'eastward'} H {'r', 'Westward', 'o', 'd', 'e'} set()
Python Set Operations
Sets can be used to carry out mathematical set operations like union, intersection, difference and symmetric departure. Nosotros can do this with operators or methods.
Let us consider the following two sets for the following operations.
>>> A = {1, two, 3, 4, 5} >>> B = {4, v, half dozen, 7, 8}
Ready Union
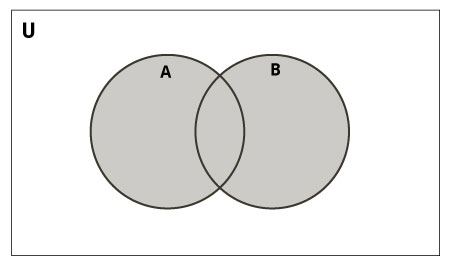
Union of A and B is a gear up of all elements from both sets.
Spousal relationship is performed using |
operator. Same tin be accomplished using the wedlock()
method.
# Set wedlock method # initialize A and B A = {1, ii, 3, 4, 5} B = {four, 5, half dozen, 7, 8} # use | operator # Output: {one, 2, 3, iv, 5, half dozen, 7, 8} impress(A | B)
Output
{1, two, three, 4, five, 6, 7, viii}
Endeavor the following examples on Python shell.
# use union function >>> A.union(B) {1, 2, 3, 4, 5, 6, seven, 8} # use union function on B >>> B.marriage(A) {one, two, 3, four, 5, half dozen, 7, viii}
Set Intersection
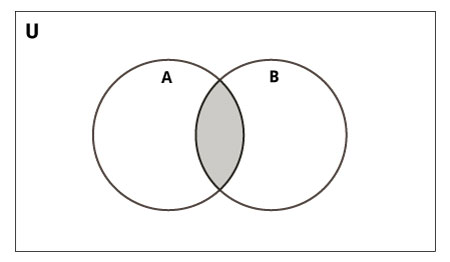
Intersection of A and B is a set of elements that are mutual in both the sets.
Intersection is performed using &
operator. Same can be accomplished using the intersection()
method.
# Intersection of sets # initialize A and B A = {1, ii, 3, iv, v} B = {4, five, vi, vii, 8} # apply & operator # Output: {4, five} impress(A & B)
Output
{iv, 5}
Try the post-obit examples on Python crush.
# utilise intersection function on A >>> A.intersection(B) {four, 5} # use intersection function on B >>> B.intersection(A) {four, 5}
Set Departure
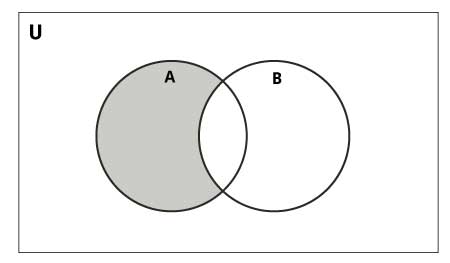
Departure of the set B from set A(A - B) is a set of elements that are only in A but non in B. Similarly, B - A is a set up of elements in B only not in A.
Difference is performed using -
operator. Same can be accomplished using the difference()
method.
# Divergence of two sets # initialize A and B A = {1, 2, iii, 4, 5} B = {4, v, vi, 7, 8} # utilize - operator on A # Output: {one, 2, 3} print(A - B)
Output
{1, 2, 3}
Try the following examples on Python shell.
# utilise difference office on A >>> A.difference(B) {1, 2, 3} # use - operator on B >>> B - A {8, 6, 7} # employ difference function on B >>> B.difference(A) {eight, 6, 7}
Gear up Symmetric Difference
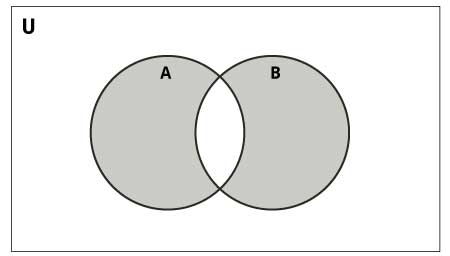
Symmetric Difference of A and B is a set of elements in A and B only not in both (excluding the intersection).
Symmetric difference is performed using ^
operator. Same can be accomplished using the method symmetric_difference()
.
# Symmetric difference of ii sets # initialize A and B A = {one, ii, iii, 4, 5} B = {four, 5, 6, vii, 8} # apply ^ operator # Output: {1, 2, 3, 6, 7, 8} print(A ^ B)
Output
{1, two, 3, vi, 7, 8}
Try the following examples on Python shell.
# use symmetric_difference office on A >>> A.symmetric_difference(B) {1, ii, 3, 6, 7, 8} # utilize symmetric_difference office on B >>> B.symmetric_difference(A) {one, 2, 3, six, 7, 8}
Other Python Set Methods
There are many set methods, some of which we have already used above. Here is a listing of all the methods that are available with the set objects:
Method | Description |
---|---|
add together() | Adds an element to the set |
clear() | Removes all elements from the set |
re-create() | Returns a re-create of the set up |
difference() | Returns the difference of 2 or more sets equally a new gear up |
difference_update() | Removes all elements of another set up from this set |
discard() | Removes an element from the set if it is a member. (Practise zilch if the chemical element is not in set) |
intersection() | Returns the intersection of two sets as a new set up |
intersection_update() | Updates the set with the intersection of itself and another |
isdisjoint() | Returns True if two sets have a cipher intersection |
issubset() | Returns True if another ready contains this set |
issuperset() | Returns True if this set contains another set |
popular() | Removes and returns an capricious set element. Raises KeyError if the fix is empty |
remove() | Removes an element from the set. If the element is not a member, raises a KeyError |
symmetric_difference() | Returns the symmetric difference of two sets equally a new set up |
symmetric_difference_update() | Updates a set with the symmetric difference of itself and another |
union() | Returns the union of sets in a new ready |
update() | Updates the set with the spousal relationship of itself and others |
Other Set Operations
Set Membership Test
We tin can test if an item exists in a set or non, using the in
keyword.
# in keyword in a set # initialize my_set my_set = set("apple") # check if 'a' is present # Output: Truthful print('a' in my_set) # check if 'p' is present # Output: False print('p' not in my_set)
Output
True False
Iterating Through a Set
Nosotros can iterate through each particular in a set using a for
loop.
>>> for letter in set("apple"): ... print(letter) ... a p east l
Born Functions with Set
Built-in functions like all()
, any()
, enumerate()
, len()
, max()
, min()
, sorted()
, sum()
etc. are normally used with sets to perform different tasks.
Function | Description |
---|---|
all() | Returns True if all elements of the set are true (or if the set is empty). |
whatever() | Returns True if whatever element of the set is true. If the set is empty, returns False . |
enumerate() | Returns an enumerate object. It contains the index and value for all the items of the set as a pair. |
len() | Returns the length (the number of items) in the set. |
max() | Returns the largest particular in the set up. |
min() | Returns the smallest detail in the set. |
sorted() | Returns a new sorted list from elements in the set up(does non sort the prepare itself). |
sum() | Returns the sum of all elements in the set. |
Python Frozenset
Frozenset is a new grade that has the characteristics of a gear up, but its elements cannot be changed once assigned. While tuples are immutable lists, frozensets are immutable sets.
Sets being mutable are unhashable, then they can't be used as dictionary keys. On the other paw, frozensets are hashable and can be used as keys to a dictionary.
Frozensets can exist created using the frozenset() function.
This data type supports methods like copy()
, difference()
, intersection()
, isdisjoint()
, issubset()
, issuperset()
, symmetric_difference()
and union()
. Being immutable, it does not take methods that add or remove elements.
# Frozensets # initialize A and B A = frozenset([ane, 2, 3, 4]) B = frozenset([3, 4, 5, 6])
Attempt these examples on Python shell.
>>> A.isdisjoint(B) False >>> A.deviation(B) frozenset({1, 2}) >>> A | B frozenset({1, 2, iii, iv, 5, 6}) >>> A.add(iii) ... AttributeError: 'frozenset' object has no attribute 'add together'
How To Add List To A Set In Python,
Source: https://www.programiz.com/python-programming/set
Posted by: tiedemancovest.blogspot.com
0 Response to "How To Add List To A Set In Python"
Post a Comment